Understanding How Many Lines a Python Program Will Print is to first understand the flow and structure of loops can help you predict how many times certain actions will be performed. In this blog post, we will break down a simple program and analyze how many lines this program will print? x = 10 while x > 0: print(x) x = x – 3, specifically focusing on a while
loop.
Let’s take a look at the Python program in question:
pythonCopy codex = 10
while x > 0:
print(x)
x = x - 3
The goal here is to determine how many lines this program will print. We will walk through each step, line by line, and understand how the program works to reach the answer.
Key Concepts in the Program
Before we delve into the details, let’s break down the basic concepts used in the code.
- Variable Assignment:
- The variable
x
is initialized with the value10
. This will be the starting point for the loop.
- The variable
- While Loop:
- The
while
loop checks if the condition (x > 0
) is true. If it is, the program will execute the code inside the loop body.
- The
- Print Statement:
- Every time the loop runs, it will print the current value of
x
.
- Every time the loop runs, it will print the current value of
- Decrement Operation:
- After each iteration, the value of
x
is decreased by 3 (x = x - 3
). This ensures that the loop doesn’t run indefinitely and will eventually stop whenx
is no longer greater than 0.
- After each iteration, the value of
Step-by-Step Breakdown of the Program
Now, let’s simulate what happens each time the loop runs to see how many lines the program will print.
- First Iteration:
x = 10
(initial value)- The
while
conditionx > 0
is true (because 10 is greater than 0), so the program enters the loop. - The program prints
10
and then subtracts 3 fromx
. Now,x = 7
.
10
- Second Iteration:
x = 7
(updated value)- The condition
x > 0
is still true (7 is greater than 0). - The program prints
7
and then subtracts 3 fromx
. Now,x = 4
.
10
,7
- Third Iteration:
x = 4
(updated value)- The condition
x > 0
is true (4 is greater than 0). - The program prints
4
and then subtracts 3 fromx
. Now,x = 1
.
10
,7
,4
- Fourth Iteration:
x = 1
(updated value)- The condition
x > 0
is still true (1 is greater than 0). - The program prints
1
and then subtracts 3 fromx
. Now,x = -2
.
10
,7
,4
,1
- Exit Condition:
x = -2
(updated value)- The condition
x > 0
is now false (because -2 is not greater than 0), so the loop terminates.
10
,7
,4
,1
How Many Lines Will It Print? x = 10 while x > 0: print(x) x = x – 3
The program prints a total of 4 lines: 10
, 7
, 4
, and 1
.
Explanation of the Output
The while
loop continues to execute as long as the condition x > 0
is true. In each iteration, the value of x
decreases by 3. The loop stops when x
becomes -2, which is the first time x
is no longer greater than 0. By counting the iterations, we can see that the program prints four lines.
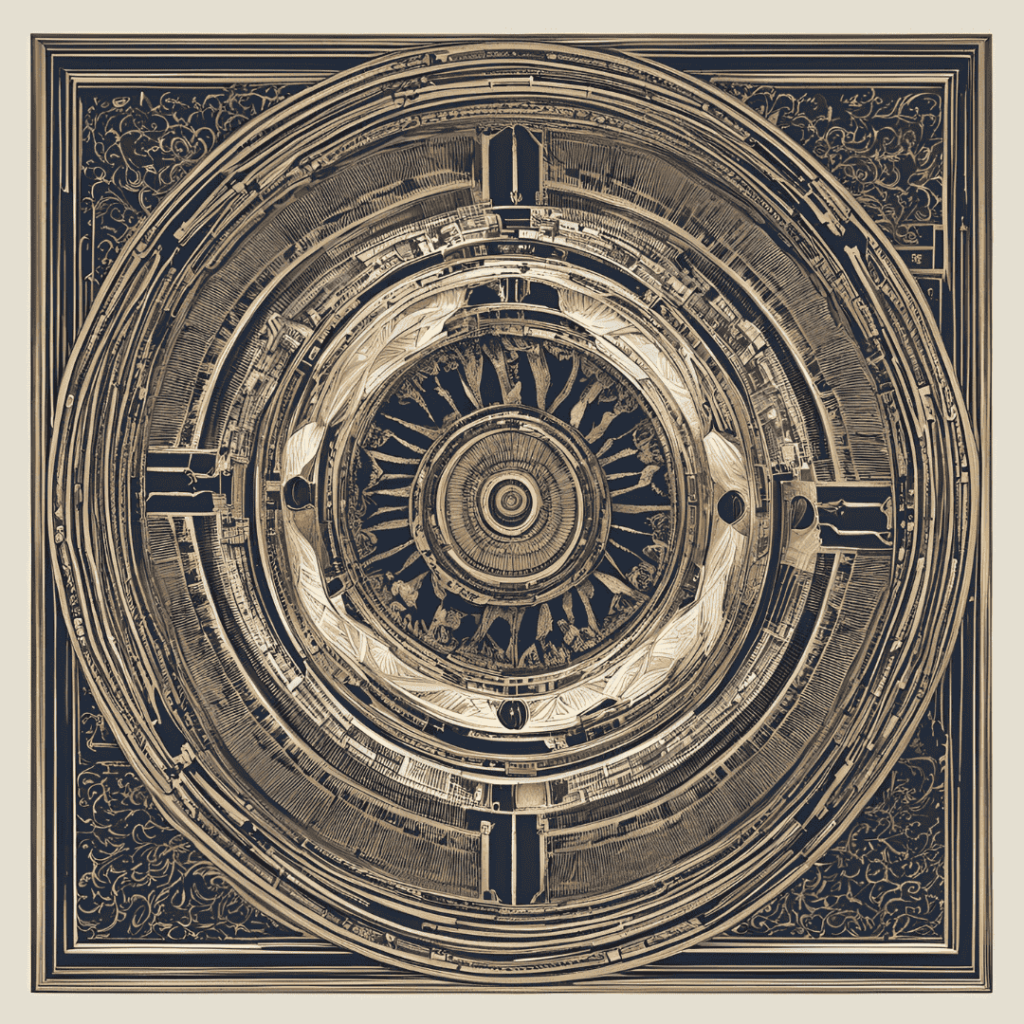
Practical Example and Considerations
If you change the initial value of x
, the number of printed lines will also change. Here’s how:
- Starting with a Larger
x
: If you initializex
with a larger value, for example,x = 20
, the program would print more lines:pythonCopy codex = 20 while x > 0: print(x) x = x - 3
Output:20
,17
,14
,11
,8
,5
,2
This results in 7 lines of output, as the loop iterates more times beforex
becomes negative. - Starting with a Smaller
x
: Ifx
starts with a smaller value, for example,x = 5
, the program will print fewer lines:pythonCopy codex = 5 while x > 0: print(x) x = x - 3
Output:5
,2
This results in just 2 lines of output.
Conclusion
The key takeaway from this example is how the loop condition and decrement operation work together to determine the number of times the loop runs. In our original case, the program printed 4 lines because the starting value of x
allowed for four iterations before x
became less than or equal to 0.
For beginners in Python, this example is a great way to understand loops and conditions. By experimenting with different starting values for x
, you can gain more insights into how loops work in real-world programming scenarios.